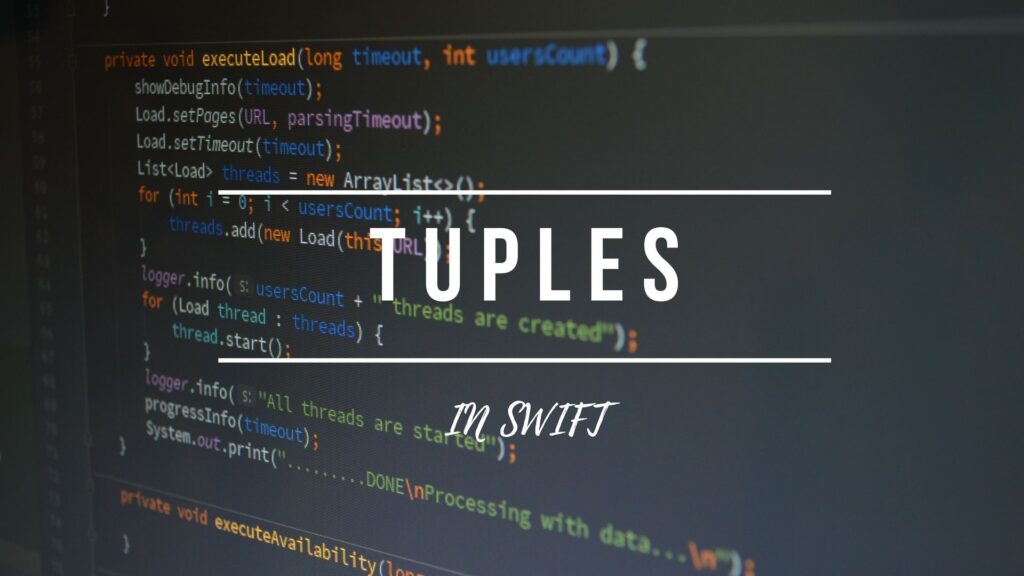
For most of the time you will be working with the single pieces of data, but sometimes data in your apps come in pairs or triplets. For example, imagine if you have a set of x, y, and z coordinates on a 3D grid. In Swift, you can represent related data like this in a simple way through the use of Tuples. By the time you’re done this exercises, you’ll know another really effective solution for storing different data types. Now let’s create our first tuple…
Creating a Tuple
let person: (String, Int) = (“Tim”, 32)
First, we defined a constant smartphone and provided the necessary types inside the parentheses separated by a comma. Since there are a String and Int we can set it to be equal to values iPhone and 8. For more convenience, we can also use type inference to shorten this statement, since the compiler can understand that we are using a tuple with a String and Integer values. Pretty cool!
let person = (“Tim”, 32)
The great thing about the tuples is that we can actually use Doubles instead of Integer and the compiler will automatically detect the type itself, which gives us the flexibility to have tuples with mixed types.
In case if you would like to check the type of the data you can option + click in the compiler and the Xcode will provide you a detailed information about the object.
Decomposing Tuples
If you are using the playgrounds in Xcode in the preview section you would be able to see the following (.0 “Tim”, .1 8) which means that .0 is the first value in the tuple and .1 is the second value of the tuple. So now we can actually use this information to access the values pretty easily.
let name = person.0
let age = person.1
Named Elements
Sometimes it can be a little bit confusing to refer to values inside a tuple by a number since it’s not immediately clear what we want to do, so instead, you can actually name each individual part inside a tuple if you would like. Here’s how you do this…
let person = (name: “Tim”, age: 32)
Now to access the first values we can simply write .name and to see the second value we use .age which actually makes the code easier to read.
let name1 = person.name
let age1 = person.age
Multiple Assignment
Now if we decide to add 3 elements to our tuple accessing all three values would be a little bit tedious right? Because we’d have to add three more lines of code, but let’s imagine if you had 20 new elements in your tuple. Well, actually there’s a shortcut if you want to pull out all of these values on a tuple into variables that you can use later. Simply just provide the values you would like to use in the parentheses.
let person = ("Tim", "Anderson", 32) let (name, surname, age) = person print(name) print(surname) print(age) // Prints Tim // Prints Anderson // Prints 32
So the following code is basically creating three variables name, surname and age for us all in one line, which we can access later in our code.
Ignoring Part of a Tuple
In case if you want to pull out the values of a tuple, but you only care about the first two values then you can add an underscore at the end to ignore the last one, while still having access to first two values.
let person = ("Tim", "Anderson", 32) let (name, surname, _) = person print(name) print(surname) // Prints Tim // Prints Anderson
Functions with Multiple Return Values
Tuples are extremely useful while working with functions since we can return multiple values.
func person() -> (name: String, age: Int) { let theName = "Tim" let theAge = 32 return (theName, theAge) } let example = person() print("My name is \(example.name) and I'm \(example.age) years old.") // Prints My name is Tim and I'm 32 years old.
The following function returns a tuple which contains String and Integer values, that can be accessed according to the label names. Since the tuple values are named as a return type, we can use the dot syntax to access those values as we did in the print statement.
Further Reading
Now you know the basics of tuples, you can simply combine different types of data together. later in this course, you’ll also learn other ways to combine values using Structures and Classes, but tuples are still great when you need to put different values together in a quick and temporary way.
Leave a Reply